NestJS WebSockets Tutorial: Building Real-Time Applications with WebSocket Integration
WebSockets have become an essential part of modern web development, enabling real-time communication between clients and servers. NestJS, a powerful NodeJs framework, offers a seamless integration of WebSockets, making it a top choice for building real-time applications. In this NestJS WebSockets tutorial, we will explore how to implement WebSockets in NestJS and create a real-time chat application as an example.
Complete source code for this nestjs websocket example is available in github repository.
Table of Contents:
Below is a demo showcasing the appearance of our nestjs websocket example:
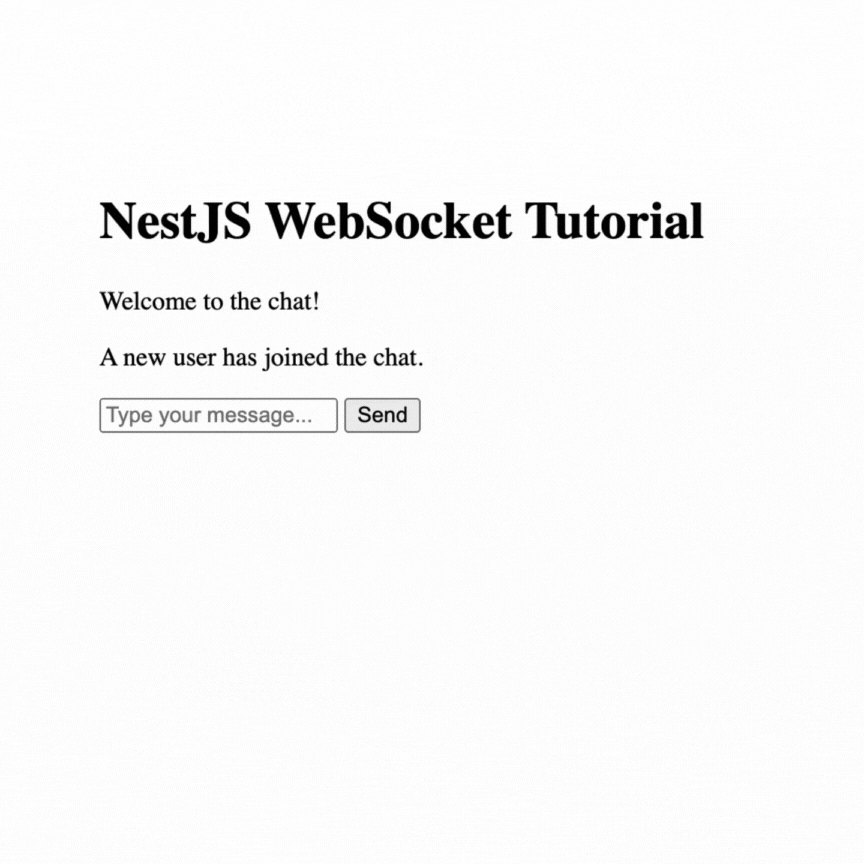
Prerequisites
Before we begin, ensure you have the following installed on your system:
- Node.js and npm (Node Package Manager)
- NestJS CLI (Command Line Interface)
Introduction to NestJS Websocket
The @nestjs/websockets
package provides various decorators and interfaces to work with WebSocket functionality in NestJS. Here is a list of some of the key methods and decorators available in @nestjs/websockets
:
@WebSocketGateway()
- Decorator used to mark a class as a WebSocket Gateway. WebSocket Gateways are responsible for handling WebSocket connections and events.
@WebSocketServer()
- Decorator used to inject the underlying
socket.io
server instance into a WebSocket Gateway. It allows you to access and interact with the raw Socket.IO server.
- Decorator used to inject the underlying
@SubscribeMessage(event: string)
- Decorator used in WebSocket Gateways to define event handlers for specific WebSocket messages. The event name specified in the decorator corresponds to the name of the WebSocket message that the handler will react to.
@MessageBody()
- Decorator used in WebSocket message handlers to extract and inject the message payload (body) into the handler function. It allows you to access the data sent by the client in the WebSocket message.
@ConnectedSocket()
- Decorator used to inject the client socket instance into a WebSocket Gateway event handler. It provides access to the client’s socket object, allowing you to perform operations directly on the client’s connection.
@OnGatewayConnection()
- Interface that defines the
handleConnection
method, called when a new client connects to the WebSocket Gateway. This method allows you to handle the connection event and perform actions when a client establishes a WebSocket connection.
- Interface that defines the
@OnGatewayDisconnect()
- Interface that defines the
handleDisconnect
method, called when a client disconnects from the WebSocket Gateway. This method allows you to handle the disconnection event and perform cleanup tasks or notify other clients about the disconnection.
- Interface that defines the
WebSocketAdapter
- The WebSocketAdapter is an abstract class that can be extended to create custom WebSocket adapters in NestJS. WebSocket adapters can be used to customize the underlying WebSocket server implementation and handle specific WebSocket events.
These are just some of the key methods and decorators available in @nestjs/websockets
. NestJS provides a robust and flexible WebSocket module that empowers developers to build real-time applications with ease. By leveraging these methods and decorators, developers can create interactive and efficient applications that deliver an exceptional user experience.
Getting Started
Let’s start by creating a new NestJS project using the NestJS CLI:
$ nest new nestjs-websocket-example
$ cd nestjs-websocket-example
$ npm install @nestjs/serve-static --save
@nestjs/serve-static package is used to serve static web pages. In this example to show websocket functionality we will implement index.html from public folder.
Setting up WebSockets Module
NestJS provides an easy-to-use WebSocket module that abstracts the underlying WebSocket implementation. To add this module to our project, run the following command:
$ npm install @nestjs/websockets @nestjs/platform-socket.io --save
This command installs the necessary dependencies for WebSocket support and the Socket.IO adapter.
Creating a WebSocket Gateway
In NestJS, WebSocket communication is managed through WebSocket Gateways. A WebSocket Gateway is responsible for handling WebSocket connections and events.
Create a new file named chat.gateway.ts
inside the src
folder and define a WebSocket Gateway class:
import { WebSocketGateway, WebSocketServer, OnGatewayConnection, OnGatewayDisconnect } from '@nestjs/websockets';
import { Server } from 'socket.io';
@WebSocketGateway()
export class ChatGateway implements OnGatewayConnection, OnGatewayDisconnect {
@WebSocketServer() server: Server;
// Implement gateway logic here
}
The @WebSocketGateway()
decorator marks this class as a WebSocket Gateway, and we implement two interfaces, OnGatewayConnection
and OnGatewayDisconnect
, to handle connection and disconnection events.
Handling WebSocket Events
Let’s add logic to handle WebSocket events in the ChatGateway
class. For our chat application, we’ll handle the following events:
- When a client connects, we’ll emit a welcome message and notify others about the new user.
- After a client sends a message, we’ll broadcast it to all connected clients.
Add the following code to the ChatGateway
class:
import { SubscribeMessage, WebSocketGateway, WebSocketServer, OnGatewayConnection, OnGatewayDisconnect } from '@nestjs/websockets';
import { Server } from 'socket.io';
@WebSocketGateway()
export class ChatGateway implements OnGatewayConnection, OnGatewayDisconnect {
@WebSocketServer() server: Server;
// Called when a client connects
handleConnection(client: any) {
this.server.emit('message', 'Welcome to the chat!');
this.server.emit('userConnected', 'A new user has joined the chat.');
}
// Called when a client disconnects
handleDisconnect(client: any) {
this.server.emit('userDisconnected', 'A user has left the chat.');
}
// Called when a client sends a message
@SubscribeMessage('message')
handleMessage(client: any, message: string) {
this.server.emit('message', message);
}
}
Integrating the WebSocket Gateway
To activate the WebSocket Gateway, we need to update the app.module.ts
file to include it in the application:
import { Module } from '@nestjs/common';
import { ChatGateway } from './chat.gateway';
@Module({
imports: [],
controllers: [],
providers: [ChatGateway],
})
export class AppModule {}
Change main.ts file as below:
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import { NestExpressApplication } from '@nestjs/platform-express';
import { join } from 'path';
async function bootstrap() {
const app = await NestFactory.create<NestExpressApplication>(AppModule);
app.useStaticAssets(join(__dirname, '..', 'public'));
await app.listen(3000);
}
bootstrap();
Creating the Frontend
Now that our WebSocket backend is set up, let’s create a simple frontend to interact with the WebSocket.
Create an index.html
file in the public
folder of the project:
<!DOCTYPE html>
<html>
<head>
<title>NestJS WebSocket Tutorial</title>
</head>
<body>
<h1>NestJS WebSocket Tutorial</h1>
<div id="chat"></div>
<input type="text" id="message" placeholder="Type your message..." />
<button onclick="sendMessage()">Send</button>
<script src="https://cdn.socket.io/4.6.0/socket.io.min.js" integrity="sha384-c79GN5VsunZvi+Q/WObgk2in0CbZsHnjEqvFxC5DxHn9lTfNce2WW6h2pH6u/kF+" crossorigin="anonymous"></script>
<script>
const socket = io('http://localhost:3000');
socket.on('message', (message) => {
const chatDiv = document.getElementById('chat');
chatDiv.innerHTML += `<p>${message}</p>`;
});
socket.on('userConnected', (message) => {
const chatDiv = document.getElementById('chat');
chatDiv.innerHTML += `<p>${message}</p>`;
});
socket.on('userDisconnected', (message) => {
const chatDiv = document.getElementById('chat');
chatDiv.innerHTML += `<p>${message}</p>`;
});
function sendMessage() {
const messageInput = document.getElementById('message');
const message = messageInput.value;
socket.emit('message', message);
messageInput.value = '';
}
</script>
</body>
</html>
Running the Application
Before we can run the application, make sure you have the NestJS server and the frontend running simultaneously. In one terminal, start the NestJS server:
$ npm run start
In another terminal, navigate to the public
folder and open the index.html
file in a web browser.
Now you should have a simple real-time chat application built with NestJS and WebSockets. When you open multiple browser windows and send messages, you’ll see the messages appearing in real-time on all connected clients.
In this tutorial, we explored how to implement WebSocket communication in NestJS to build a real-time chat application. NestJS’s WebSocket module provides a straightforward way to handle WebSocket events and enables seamless communication between clients and servers.
Remember that this is just a basic example, and you can extend this setup to create more sophisticated real-time applications, such as live notifications, real-time gaming, and collaborative editing tools. NestJS’s WebSocket support empowers you to build scalable and efficient real-time applications with ease. Happy coding!
Unlock the power of NestJS and MongoDB to create a robust and efficient RESTful API web service. Dive into this informative post to master the process.
By Kurukshetran